HTML, CSS3, JS 自适应表单场景灵活应用id1018-网页设计
Warning: Undefined property: stdClass::$maxbutton id="5" in /www/wwwroot/code.5g-o.com/wp-content/plugins/kama-clic-counter/class.KCCounter.php on line 733
<!doctype html> <html lang="zh"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"> <meta name="viewport" content="width=device-width, initial-scale=1.0 maximum-scale=1.0, user-scalable=no"/> <title>jQuery响应式表格插件basictable </title> <link rel="stylesheet" type="text/css" href="https://code.5g-o.com/wp-content/uploads/2020/12/demo.css"> <link rel="stylesheet" type="text/css" href="https://code.5g-o.com/wp-content/uploads/2020/12/style.css" /> <link rel="stylesheet" type="text/css" href="https://code.5g-o.com/wp-content/uploads/2020/12/basictable.css" /> </head> <body> <div class="container"> <div id="page"> <h1>基本表格Demo</h1> <h2>基本实现</h2> <p>基本实现使用默认参数设置。表格的响应式效果发生在宽度小于等于568像素的时候。</p> <table id="table"> <thead> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> <th>Height</th> <th>Province</th> <th>Sport</th> </tr> </thead> <tbody> <tr> <td>Jill Smith</td> <td>25</td> <td>Female</td> <td>5'4</td> <td>British Columbia</td> <td>Volleyball</td> </tr> <tr> <td>John Stone</td> <td>30</td> <td>Male</td> <td>5'9</td> <td>Ontario</td> <td>Badminton</td> </tr> <tr> <td>Jane Strip</td> <td>29</td> <td>Female</td> <td>5'6</td> <td>Manitoba</td> <td>Hockey</td> </tr> <tr> <td>Gary Mountain</td> <td>21</td> <td>Mail</td> <td>5'8</td> <td>Alberta</td> <td>Curling</td> </tr> <tr> <td>James Camera</td> <td>31</td> <td>Male</td> <td>6'1</td> <td>British Columbia</td> <td>Hiking</td> </tr> </tbody> </table> <code class="js"> $('#table').basictable(); </code> <h2>修改Breakpoint</h2> <p>你可以手动修改每个表格的breakpoint。下面的例子将表格的breakpoint设置为768像素,以适应典型的平板电脑和portrait模式。</p> <table id="table-breakpoint"> <thead> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> <th>Height</th> <th>Province</th> <th>Sport</th> </tr> </thead> <tbody> <tr> <td>Jill Smith</td> <td>25</td> <td>Female</td> <td>5'4</td> <td>British Columbia</td> <td>Volleyball</td> </tr> <tr> <td>John Stone</td> <td>30</td> <td>Male</td> <td>5'9</td> <td>Ontario</td> <td>Badminton</td> </tr> <tr> <td>Jane Strip</td> <td>29</td> <td>Female</td> <td>5'6</td> <td>Manitoba</td> <td>Hockey</td> </tr> <tr> <td>Gary Mountain</td> <td>21</td> <td>Mail</td> <td>5'8</td> <td>Alberta</td> <td>Curling</td> </tr> <tr> <td>James Camera</td> <td>31</td> <td>Male</td> <td>6'1</td> <td>British Columbia</td> <td>Hiking</td> </tr> </tbody> </table> <code class="js"> $('#table-breakpoint').basictable({<br /> breakpoint: 768,<br /> }); </code> <h2>Force Responsive Off</h2> <p>The script will not force the table to be responsive. The table will only go into responsive mode when the table is actually larger than its parent container. In this demo the parent of table is the div with id page.</p> <table id="table-force-off"> <thead> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> <th>Height</th> <th>Province</th> <th>Sport</th> </tr> </thead> <tbody> <tr> <td>Jill Smith</td> <td>25</td> <td>Female</td> <td>5'4</td> <td>British Columbia</td> <td>Volleyball</td> </tr> <tr> <td>John Stone</td> <td>30</td> <td>Male</td> <td>5'9</td> <td>Ontario</td> <td>Badminton</td> </tr> <tr> <td>Jane Strip</td> <td>29</td> <td>Female</td> <td>5'6</td> <td>Manitoba</td> <td>Hockey</td> </tr> <tr> <td>Gary Mountain</td> <td>21</td> <td>Mail</td> <td>5'8</td> <td>Alberta</td> <td>Curling</td> </tr> <tr> <td>James Camera</td> <td>31</td> <td>Male</td> <td>6'1</td> <td>British Columbia</td> <td>Hiking</td> </tr> </tbody> </table> <code class="js"> $('#table-force-off').basictable({<br /> forceResponsive: false,<br /> }); </code> <h2>最大高度</h2> <p>Some tables could get very long in responsive. You could set a max-height in mobile where scrolling within the table would happen. Turn on tableWrapper to get a container around the table that toggles an active class. You could also just create your own wrapper and match the breakpoint to do this.</p> <table id="table-max-height" class="max-height"> <thead> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> <th>Height</th> <th>Province</th> <th>Sport</th> </tr> </thead> <tbody> <tr> <td>Jill Smith</td> <td>25</td> <td>Female</td> <td>5'4</td> <td>British Columbia</td> <td>Volleyball</td> </tr> <tr> <td>John Stone</td> <td>30</td> <td>Male</td> <td>5'9</td> <td>Ontario</td> <td>Badminton</td> </tr> <tr> <td>Jane Strip</td> <td>29</td> <td>Female</td> <td>5'6</td> <td>Manitoba</td> <td>Hockey</td> </tr> <tr> <td>Gary Mountain</td> <td>21</td> <td>Mail</td> <td>5'8</td> <td>Alberta</td> <td>Curling</td> </tr> <tr> <td>James Camera</td> <td>31</td> <td>Male</td> <td>6'1</td> <td>British Columbia</td> <td>Hiking</td> </tr> </tbody> </table> <code class="js"> $('#table-max-height').basictable({<br /> tableWrapper: true<br /> }); </code> <h2>CSS Controls</h2> <h3>Use Media Query Over JS Resize</h3> <p>If you don't want to use the js bind on resize you can use css to control the breakpoint itself. Using basic table to setup the structure itself and copying basictable.css styles into your own media query.</p> <table id="table-no-resize"> <thead> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> <th>Height</th> <th>Province</th> <th>Sport</th> </tr> </thead> <tbody> <tr> <td>Jill Smith</td> <td>25</td> <td>Female</td> <td>5'4</td> <td>British Columbia</td> <td>Volleyball</td> </tr> <tr> <td>John Stone</td> <td>30</td> <td>Male</td> <td>5'9</td> <td>Ontario</td> <td>Badminton</td> </tr> <tr> <td>Jane Strip</td> <td>29</td> <td>Female</td> <td>5'6</td> <td>Manitoba</td> <td>Hockey</td> </tr> <tr> <td>Gary Mountain</td> <td>21</td> <td>Mail</td> <td>5'8</td> <td>Alberta</td> <td>Curling</td> </tr> <tr> <td>James Camera</td> <td>31</td> <td>Male</td> <td>6'1</td> <td>British Columbia</td> <td>Hiking</td> </tr> </tbody> </table> <code class="css"> @media only screen and (max-width: 568px) {<br /> #table-no-resize thead {<br /> display: none;<br /> }<br /><br /> #table-no-resize tbody td {<br /> border: none !important;<br /> display: block;<br /> vertical-align: top;<br /> }<br /><br /> #table-no-resize tbody td:before {<br /> content: attr(data-th) ": ";<br /> display: inline-block;<br /> font-weight: bold;<br /> width: 6.5em;<br /> }<br /><br /> #table-no-resize tbody td.bt-hide {<br /> display: none;<br /> }<br /> } </code> <code class="js"> $('#table-no-resize').basictable({<br /> noResize: true,<br /> }); </code> <h3>Two Axis Styling</h3> <p>Two axis could be styled differently. This does not need to be done through the library itself. The example code will show how to target the first column in desktop and first row in responsive mode.</p> <table id="table-two-axis" class="two-axis"> <thead> <tr> <th>Name</th> <th>Age</th> <th>Gender</th> <th>Height</th> <th>Province</th> <th>Sport</th> </tr> </thead> <tbody> <tr> <td>Jill Smith</td> <td>25</td> <td>Female</td> <td>5'4</td> <td>British Columbia</td> <td>Volleyball</td> </tr> <tr> <td>John Stone</td> <td>30</td> <td>Male</td> <td>5'9</td> <td>Ontario</td> <td>Badminton</td> </tr> <tr> <td>Jane Strip</td> <td>29</td> <td>Female</td> <td>5'6</td> <td>Manitoba</td> <td>Hockey</td> </tr> <tr> <td>Gary Mountain</td> <td>21</td> <td>Mail</td> <td>5'8</td> <td>Alberta</td> <td>Curling</td> </tr> <tr> <td>James Camera</td> <td>31</td> <td>Male</td> <td>6'1</td> <td>British Columbia</td> <td>Hiking</td> </tr> </tbody> </table> <code class="css"> table.two-axis tr td:first-of-type {<br /> background: #dff1f7;<br /> }<br /><br /> @media only screen and (max-width: 568px) {<br /> table.two-axis tr td:first-of-type,<br /> table.two-axis tr:nth-of-type(2n+2) td:first-of-type,<br /> table.two-axis tr td:first-of-type:before {<br /> background: #dff1f7;<br /> color: #ffffff;<br /> }<br /><br /> table.two-axis tr td:first-of-type {<br /> border-bottom: 1px solid #e4ebeb;<br /> }<br /> } </code> </div> </div> basictable.css <script src="https://code.5g-o.com/wp-content/uploads/2020/12/jquery-2.1.1.min.js" type="text/javascript"></script> <script type="text/javascript" src="https://code.5g-o.com/wp-content/uploads/2020/12/jquery.basictable.min.js"></script> <script type="text/javascript"> $(document).ready(function() { $('#table').basictable(); $('#table-breakpoint').basictable({ breakpoint: 768 }); $('#table-swap-axis').basictable({ swapAxis: true }); $('#table-force-off').basictable({ forceResponsive: true }); $('#table-no-resize').basictable({ noResize: true }); $('#table-two-axis').basictable(); $('#table-max-height').basictable({ tableWrapper: true }); }); </script> </body> </html>
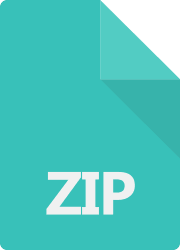
一键获取本网站前端代码设计的所有源码
获取资源构建和完善自己的源码库
源码可以在本地直接演示
同时研究和体验 如何将一些具体的想法的实现过程
源码可以直接嫁接到自己的网站里复用
稍作修改成为自己的作品